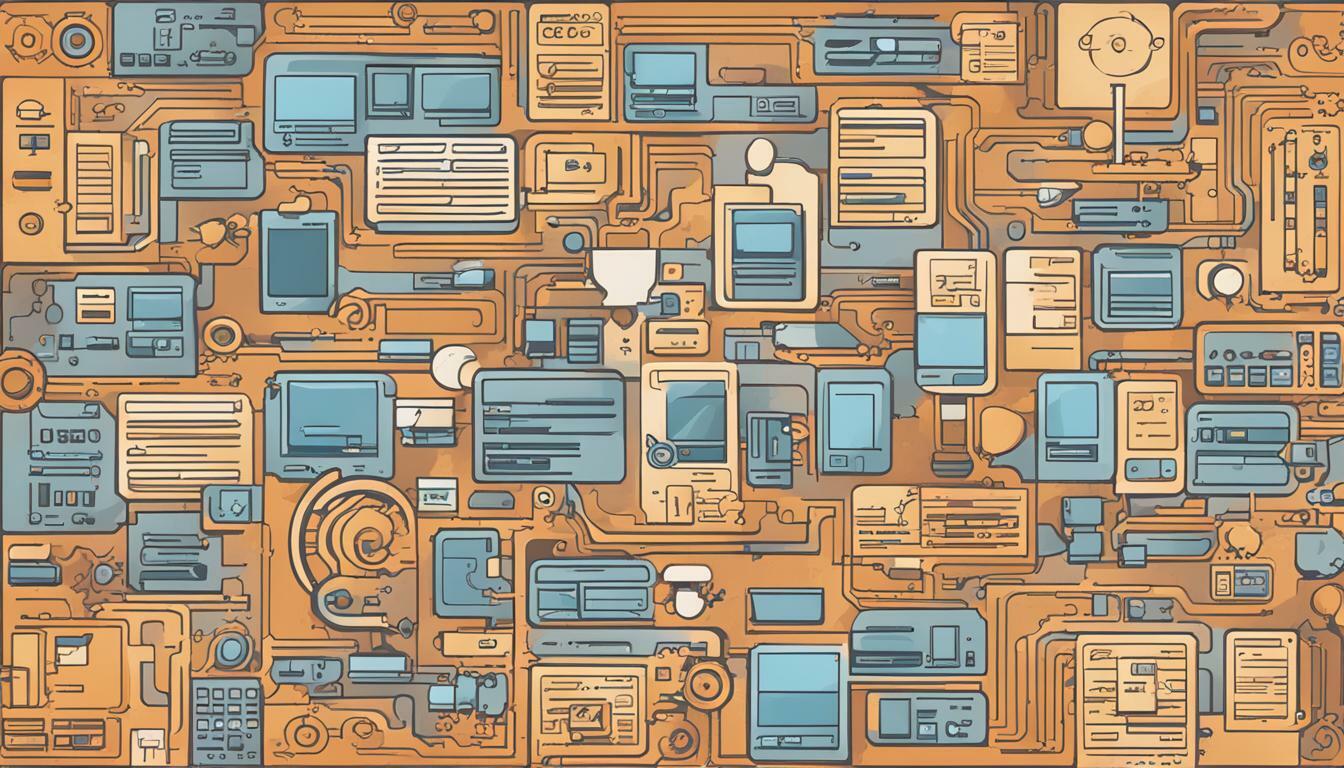
Welcome to our comprehensive guide on asynchronous programming in C# and its role in enhancing API responsiveness. In today’s fast-paced digital world, efficient API performance is essential for businesses to stay competitive and meet user demands. Asynchronous programming can play a crucial role in achieving this by allowing API calls to execute concurrently and avoiding blocking operations.
In this article, we will explain the concept of asynchronous programming in C#, its benefits for API responsiveness, common challenges and best practices, and tools and libraries available for developers. Our goal is to equip you with the knowledge and resources needed to write robust, responsive, and high-performing APIs using asynchronous programming in C#.
Key Takeaways:
- Asynchronous programming in C# can significantly improve API responsiveness by allowing concurrent execution and avoiding blocking operations.
- Understanding async and await keywords in C# is essential for writing efficient and responsive code.
- Designing asynchronous APIs that prioritize responsiveness requires careful consideration of return types, progress reporting, and cancellation mechanisms.
- Common challenges in asynchronous programming, such as exception handling and resource management, can be overcome with best practices and techniques.
- Various tools and libraries are available for asynchronous programming in C#, including the Task Parallel Library and async-await pattern.
Understanding Asynchronous Programming in C#
Asynchronous programming is a technique used to optimize API performance by enabling concurrent execution of multiple operations. Unlike synchronous programming, where the program executes sequentially, asynchronous programming allows the program to execute multiple tasks simultaneously, improving overall efficiency and responsiveness.
In C#, asynchronous programming is implemented using the async and await keywords. The async keyword indicates that a method is asynchronous, while the await keyword allows the program to continue execution while the asynchronous operation completes.
When writing asynchronous code, it is essential to follow best practices to ensure optimal performance. This includes avoiding blocking operations, using cancellation tokens to stop long-running operations, and properly handling exceptions.
By using asynchronous programming, developers can improve API responsiveness, reduce latency, and enhance user experience. However, it is critical to understand the fundamentals and best practices of async programming in C# to achieve these benefits.
Benefits of Asynchronous Programming for API Responsiveness
When it comes to building APIs that are both efficient and responsive, asynchronous programming can be a game changer. By enabling developers to write code that can run concurrently, async methods help reduce response times and improve overall performance. Let’s explore some of the key advantages of using async programming for API responsiveness.
Concurrent Execution
One of the most significant benefits of using asynchronous programming for API development is the ability to execute multiple operations concurrently. Rather than waiting for a single operation to complete before moving on to the next, async methods allow developers to perform multiple tasks simultaneously, significantly reducing response times.
For example, when retrieving data from a database, an async method can initiate several database calls simultaneously, allowing the CPU to work on other tasks while waiting for the results. This means that even when one operation is taking longer than expected, the API can still respond to other requests, ensuring optimal performance and responsiveness.
Avoiding Blocking Operations
Another significant advantage of async programming is the ability to avoid blocking operations that can slow down API performance. In synchronous programming, when a thread is blocked while waiting for an operation to complete, it cannot perform any other tasks, creating unnecessary delays.
With async programming, however, operations can be executed without blocking, allowing threads to continue performing other tasks while waiting for the operation to complete. This helps reduce response times and keeps the API running smoothly.
Efficient Resource Utilization
Async programming can also help optimize resource utilization by enabling the API to use system resources more efficiently. For example, when handling large file uploads, async methods can stream data as it becomes available, minimizing the amount of memory needed to process the request.
By utilizing resources efficiently, async programming can help reduce server load and improve overall performance, ensuring that the API remains responsive even under heavy traffic or load.
Real-World Scenarios
There are several real-world scenarios where async programming can make a significant difference in API responsiveness. For example, in web applications, async methods can be used to handle multiple user requests concurrently, ensuring that the application remains responsive and doesn’t become bogged down with traffic.
Similarly, in IoT applications where data is obtained from multiple sensors, async programming can help process data from each sensor concurrently, allowing for more efficient use of resources and faster response times.
As we can see, there are many benefits to using asynchronous programming for API development, ranging from faster response times to more efficient resource utilization. By following best practices and utilizing tools and libraries designed for async programming, developers can ensure that their APIs are both high-performing and responsive.
Common Challenges and Pitfalls in Asynchronous Programming
Asynchronous coding in C# can be a powerful tool for improving API responsiveness, but it also comes with its own set of challenges and pitfalls. These are some of the common issues that developers may encounter when writing asynchronous code:
- Handling Exceptions: Asynchronous code can be more difficult to debug than synchronous code. When an exception occurs, the stack trace may not provide enough information to determine the root cause. It’s important to use try-catch blocks and handle exceptions appropriately to avoid unexpected behavior.
- Managing Resources: Asynchronous operations can consume significant resources, such as memory and CPU usage. Developers need to be aware of the resources their code is using and ensure that they are not causing performance issues or blocking other operations.
- Coordinating Multiple Async Operations: When working with multiple asynchronous operations, it can be challenging to coordinate their completion. Developers need to ensure that they are using the appropriate mechanisms for coordinating and synchronizing operations to avoid deadlocks or race conditions.
Despite these challenges, with careful planning and implementation, it is possible to write robust and efficient asynchronous code that can significantly improve API responsiveness.
Best Practices for Designing Asynchronous APIs
Designing asynchronous APIs is crucial for ensuring optimal API responsiveness. Below are best practices to help developers achieve this:
1. Choose Appropriate Return Types
When designing asynchronous methods, it’s important to choose return types that accurately reflect the result of the operation. For example, tasks that return a single result should use the Task<T> class, while tasks that return multiple results should use IAsyncEnumerable<T>.
2. Provide Progress Reporting
Users of asynchronous APIs often need to track the progress of a long-running operation. Developers should provide a way to report progress updates to users, either through callback methods or events. This allows users to monitor the status of the operation and take appropriate action.
3. Design Cancellation Mechanisms
Asynchronous operations may need to be cancelled by users or system administrators. Developers should design cancellation mechanisms that enable cancellation of long-running operations without adversely affecting other tasks or threads.
4. Use Async Methods When Appropriate
Asynchronous methods should be used when performing I/O-bound operations such as calling web services or accessing databases. This frees up the calling thread to perform other operations and improves overall API responsiveness.
5. Avoid Blocking Operations
Blocking operations should be avoided in asynchronous methods as they can cause delays and adversely affect API responsiveness. Instead, developers should use non-blocking operations such as Task.WhenAll to perform multiple tasks concurrently.
By following these best practices, developers can create high-performing and user-friendly asynchronous APIs that deliver optimal API responsiveness and a great user experience.
Tools and Libraries for Asynchronous Programming in C#
If you’re looking to improve the performance and responsiveness of your C# API, asynchronous programming is a must. Fortunately, there are many tools and libraries available to help you write efficient and scalable async code. Let’s take a look at some of the most popular options.
Task Parallel Library (TPL)
TPL is a built-in framework in .NET that simplifies the process of writing asynchronous code. It provides a set of classes and methods that allow for easy parallelization of tasks, as well as coordination and synchronization of async operations. TPL is a powerful tool for improving API responsiveness.
Async-Await Pattern
Async-await is a pattern introduced in C# 5.0 that allows for the creation of asynchronous methods using keywords async and await. This pattern simplifies the process of writing async code by allowing developers to write code that looks synchronous, while still executing asynchronously. Async-await is a popular choice for writing asynchronous code in C#.
Reactive Extensions (Rx)
Rx is a library that provides a set of tools for working with asynchronous data streams in C#. It allows for the creation of reactive, event-driven programs that respond to changes in data streams. Rx can be used to create scalable and responsive APIs that handle large volumes of data.
DotNetty
DotNetty is a high-performance networking library for C# that provides a set of asynchronous networking primitives. It is designed to handle large volumes of data and support high concurrency. DotNetty is a great choice for building responsive and scalable network applications.
These are just a few of the many tools and libraries available for asynchronous programming in C#. By leveraging these tools and following best practices for async coding, you can create high-performing APIs that provide a seamless user experience.
Conclusion
Asynchronous programming is a powerful tool for enhancing API responsiveness in C#. By allowing concurrent execution, avoiding blocking operations, and utilizing system resources efficiently, async methods can greatly improve overall performance.
Developers should follow best practices when writing asynchronous code, including managing resources and coordinating multiple async operations. Additionally, designing asynchronous APIs that prioritize responsiveness is crucial for creating high-performing and user-friendly applications.
Fortunately, there are several popular tools and libraries available for asynchronous programming in C#, including Task Parallel Library (TPL) and async-await patterns. By selecting the most suitable options for their projects, developers can optimize their code and deliver performant APIs.
Overall, implementing asynchronous programming in C# can significantly enhance API responsiveness and improve the user experience. By following the best practices and utilizing the appropriate tools and libraries, developers can unlock the full potential of asynchronous programming and elevate their code to the next level.
FAQ
Q: What is asynchronous programming in C#?
A: Asynchronous programming in C# is a technique that allows code to execute concurrently, improving the responsiveness of APIs. It enables non-blocking operations and efficient utilization of system resources.
Q: How is asynchronous programming different from synchronous programming?
A: Synchronous programming executes code sequentially, blocking the program until an operation completes. Asynchronous programming, on the other hand, allows multiple tasks to execute concurrently, freeing up the program to perform other operations while waiting for tasks to complete.
Q: What are the benefits of asynchronous programming for API responsiveness?
A: Asynchronous programming can significantly enhance API responsiveness by allowing concurrent execution, avoiding blocking operations, and utilizing system resources efficiently. It improves overall performance and ensures a better user experience.
Q: What are some common challenges in asynchronous programming?
A: Challenges in asynchronous programming include handling exceptions, managing resources, and coordinating multiple async operations. Developers need to be mindful of these challenges and apply best practices to write robust asynchronous code.
Q: What are the best practices for designing asynchronous APIs?
A: When designing asynchronous APIs, it is important to choose appropriate return types, provide progress reporting, and design cancellation mechanisms. Following these best practices helps create high-performing and user-friendly APIs.
Q: Are there any tools and libraries available for asynchronous programming in C#?
A: Yes, there are popular tools and libraries such as the Task Parallel Library (TPL) and the async-await pattern that aid in asynchronous programming in C#. These tools provide various benefits and can be used in different scenarios based on project requirements.